Video game controller
Build your own gaming controller for NBA 2K23
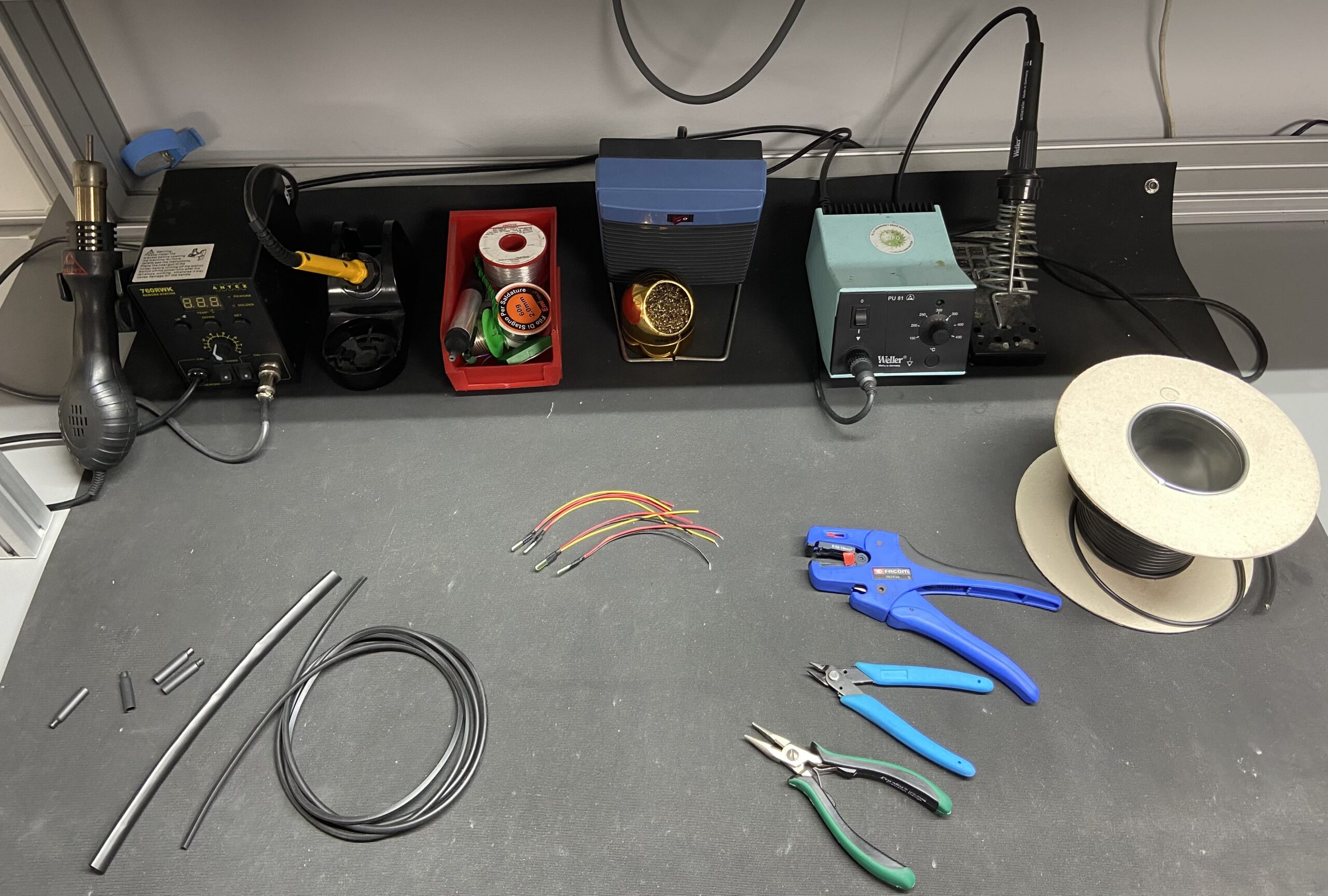
Components
2 ESP32
1 Power Supply
Supplies
Wires
Wire Striper Tool
5 Elastrolink Vein
USB Cable
Cutting Pliers
1 MPU6050
Bakelite circuit board
Soldering Iron Station
Step 1 : Sensor Test
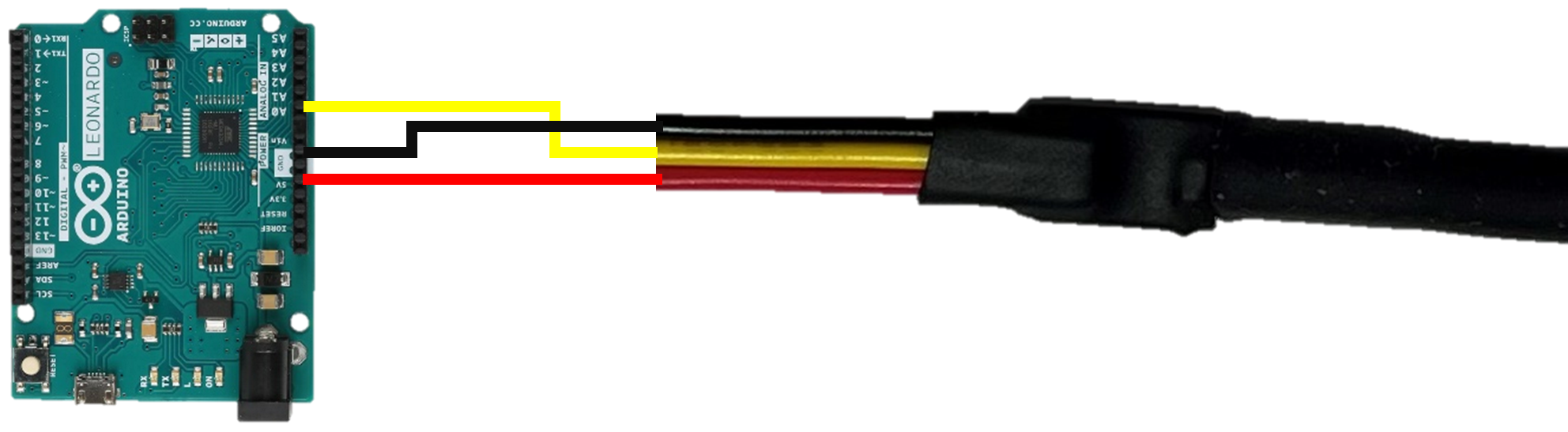
Connect the DATA wire (yellow) to A0, the red to 5V and the black one to GND
void setup() {
Serial.begin(9600);
}
Vois loop (){
Serial.println(analogRead(A0));
}
This sketch will allow you to check if your vein is working correctly.
When you’re not touching the vein it should print around 1024.
After that you can pinch the end of the vein and store the result then do the same thing with the beginning. Like that you will know the limit of your sensor.
Step 2 : Reel circuit
Circuit diagram
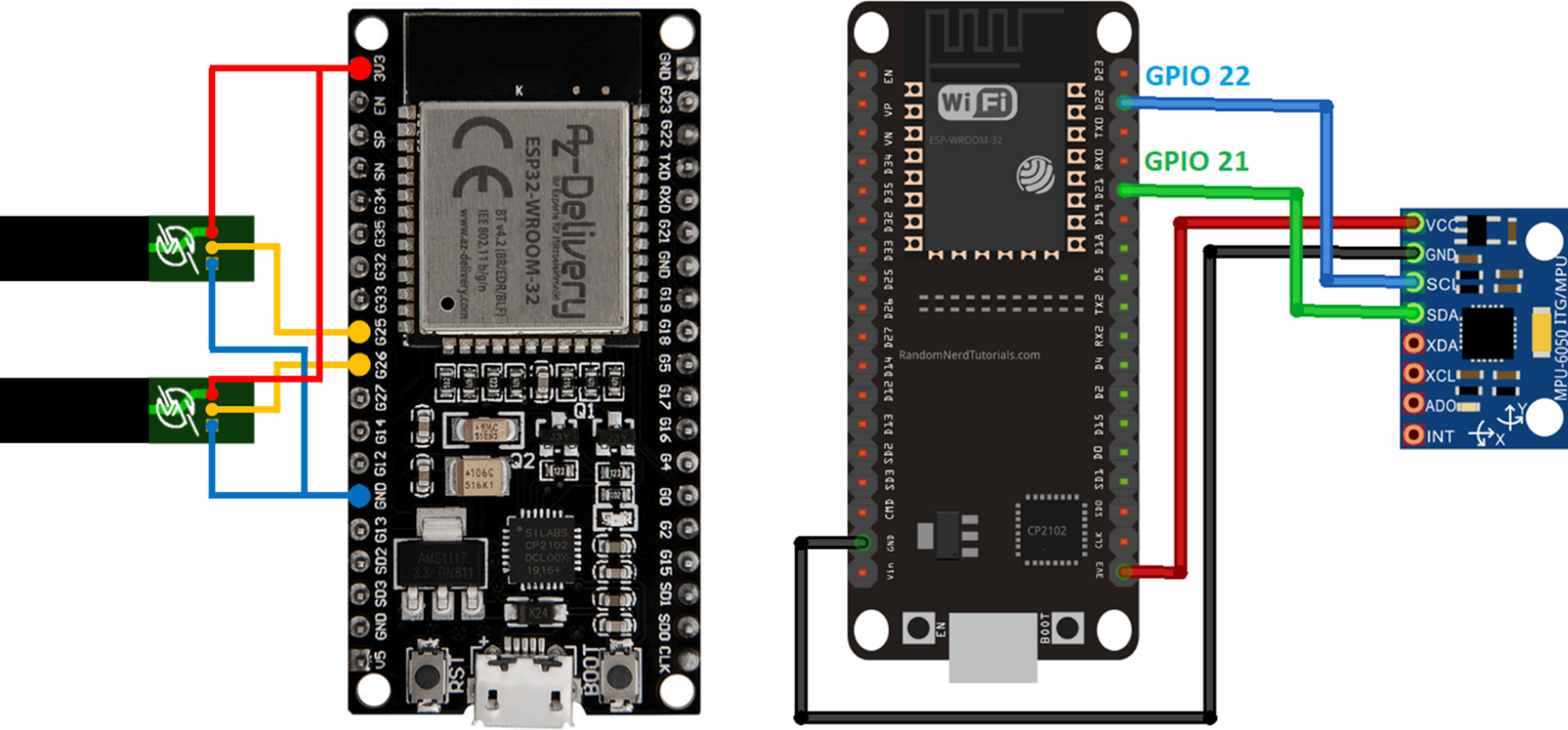
For the pad the five Elastrolink sensors are connected to A0 and A1
For the controller one MPU6050 will be connected
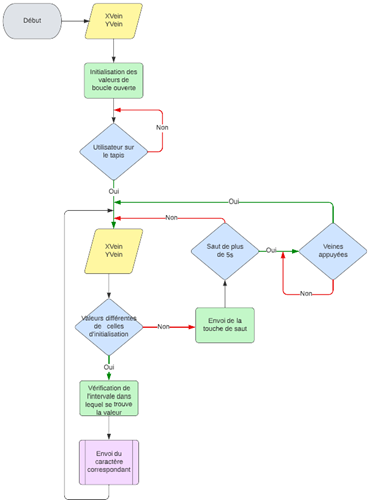
The pad
The pad is made of five Elastrolink sensors, four of 10 meters and one 3 meters
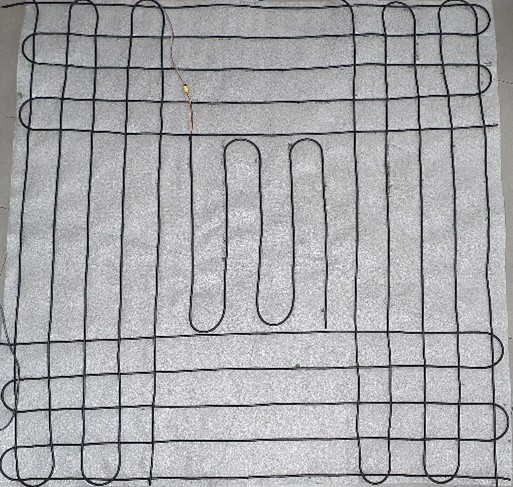
The objective is to have 9 distinct areas. In the four corner we’ve got the overlapped vein. The four side we only have one vein for each side and one sensor the center of the pad
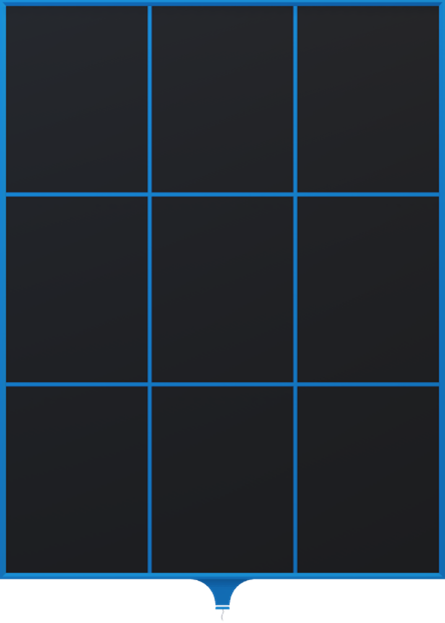
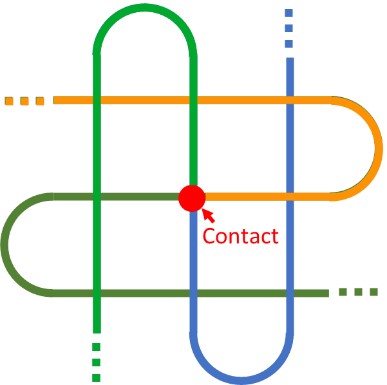
Pad program
#include “Keyboard.h”
int Z = A0;
int Q = A1;
int S = A2;
int D = A3;
int C = A4;
void setup() {
Serial.begin(9800);
Keyboard.begin();
pinMode(Z, INPUT);
pinMode(Q, INPUT);
pinMode(S, INPUT);
pinMode(D, INPUT);
pinMode(C, INPUT);
}
void loop() {
/* —– Vérification de l’appuis des diagonales —– */
if (analogRead(Z) < 800 && analogRead(Q) < 800) {
/*Si le diagonale est préssée
envoyer les lettres correspondantes et relacher les autres*/
Keyboard.press(‘z’);
Keyboard.press(‘q’);
Keyboard.release(‘s’);
Keyboard.release(‘d’);
} else if (analogRead(Z) < 800 && analogRead(D) < 800) {
Keyboard.press(‘z’);
Keyboard.press(‘d’);
Keyboard.release(‘s’);
Keyboard.release(‘q’);
} else if (analogRead(S) < 800 && analogRead(Q) < 800) {
Keyboard.press(‘s’);
Keyboard.press(‘q’);
Keyboard.release(‘z’);
Keyboard.release(‘d’);
} else if (analogRead(S) < 800 && analogRead(D) < 800) {
Keyboard.press(‘s’);
Keyboard.press(‘d’);
Keyboard.release(‘z’);
Keyboard.release(‘q’);
} else {
/* —– Vérification de l’appuis des diagonales —– */
if (analogRead(Z) < 800) {
/*Si un point cardinal est préssé
envoyer les lettres correspondantes et relacher les autres*/
Keyboard.press(‘z’);
Keyboard.release(‘q’);
Keyboard.release(‘s’);
Keyboard.release(‘d’);
} else if (analogRead(Q) < 800) {
Keyboard.release(‘z’);
Keyboard.press(‘q’);
Keyboard.release(‘s’);
Keyboard.release(‘d’);
} else if (analogRead(S) < 800) {
Keyboard.release(‘z’);
Keyboard.release(‘q’);
Keyboard.press(‘s’);
Keyboard.release(‘d’);
} else if (analogRead(D) < 800) {
Keyboard.release(‘z’);
Keyboard.release(‘q’);
Keyboard.release(‘s’);
Keyboard.press(‘d’);
} else {
Keyboard.releaseAll();
}
}
}
Controller program
The ESP32 is connected to the inertial central (MP6050) which is going to detect you trying to shoot to the basket by raising your arms. We will also be able to detect when you are faking. Because when you will shoot you will have to jump and raise your arms. If you only use your arms you will fake.
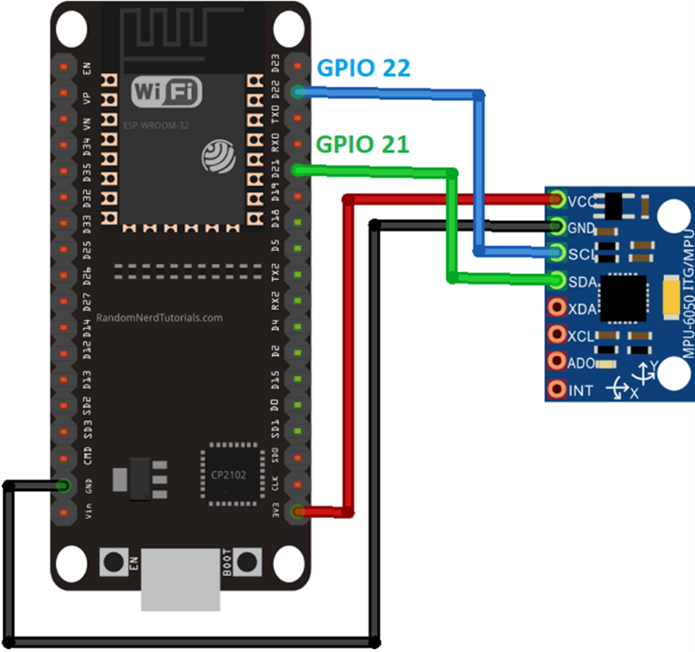
#include <BleCombo.h>
#include <BleComboKeyboard.h>
#include <BleComboMouse.h>
#include <BleConnectionStatus.h>
#include <KeyboardOutputCallbacks.h>
#include <MPU6050_tockn.h>
#include <Wire.h>
MPU6050 mpu6050(Wire);
void setup() {
Serial.begin(9600);
Wire.begin();
Keyboard.begin();
Mouse.begin();
mpu6050.begin();
mpu6050.calcGyroOffsets(true);
}
void loop() {
mpu6050.update();
if (mpu6050.getAccZ() > 1.9){
Keyboard.press(‘k’);
delay(700);
Keyboard.release(‘k’);
}
if (mpu6050.getAccX() < -1.00 || mpu6050.getAccY() > 1.00){
Keyboard.press(‘l’);
delay(200);
Keyboard.release(‘l’);
}
Serial.print(“accX : “);Serial.print(mpu6050.getAccX());
Serial.print(“\taccY : “);Serial.print(mpu6050.getAccY());
Serial.print(“\taccZ : “);Serial.println(mpu6050.getAccZ());
}